Background
Recently I’ve been working on a project to help partners demo their HoloLens solutions to potential customers. When it comes to demos, we obviously want the process to be friction-free and as painless possible. Ideally the customer could put on the device, tap an icon, and hop right into the scenario we’d like them to try. But in order to make that happen, we need to think up front about the configuration data required to make the demo function.
Here are some common questions that any demo might consider:
- How does the app know it’s running in demo mode?
- How can we skip login and go straight to the action?
- What user name and password should be used?
- What cloud service API keys should be used?
- How do we ensure these values only get used in a special demo build of the project?
- How do we avoid checking passwords and API keys into source control?
Though the answers to these questions will vary, clearly apps need a way to store configuration data that is specific to demo mode.
Sample on GitHub
I thought about how I might architect this as simply as possible. I wanted to build something ISVs might even consider dropping into their solution.
You can check out my sample project here:
Once you’ve cloned the repo, find the Unity project in the HEKDemoUnity folder.
How to use
When you first run the app you’ll see this message in the Unity console window: “No demo configuration found. Using regular app flow.”

This is because the project hasn’t been configured for demo mode. To do this, right-click on the Assets folder and choose Create -> Demo -> My Demo Data.

Give the file a name like ‘My Demo Data‘ and press enter to create it. Once the file’s created, select the file and you can now set configuration data like User Name and Password.
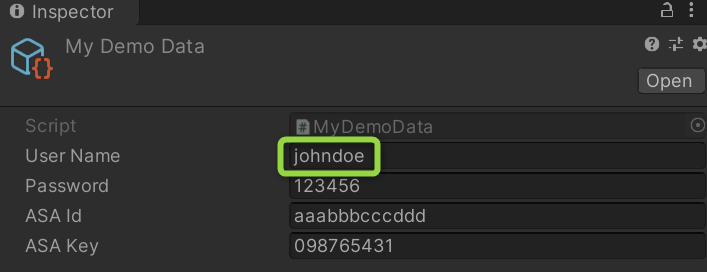
When you run the project again, you’ll see that the console window updates to show the new configuration.

How to customize
Customization is simple. Here are the steps:
- Each application would create its own class that inherits from DemoData. Check out MyDemoData as the included example.
- Configuration settings like User Name, Password and API keys can be added as public fields to your own demo data class.
- Don’t forget to add the CreateAssetMenu attribute to your own demo data class. This attribute is what allows your demo data to be created using the Unity context menu.
- Lastly all file types that represent demo data should be excluded from source control. For example, my sample .gitignore has an entry to exclude *Demo Data.*.
How it works
Because DemoData inherits from ScriptableObject, demo data classes live at the project level and don’t need to be tied to a given scene. Inheriting from ScriptableObject also makes these files optional. Your project can run with or without them.
At runtime we can tell if if the app is in demo mode simply by checking to see if demo data was included. This is as easy as reading the .Instance
property of your demo data class. If the .Instance
property returns null
, no demo data was compiled into the app. If the .Instance
property is not null
, your demo configuration is ready to be used.
Here’s a snippet of how to use demo data from the AppManager example class:
/// <summary>
/// Loads configuration data for the application.
/// </summary>
private void LoadConfiguration()
{
// If there's a demo config in the project, get a reference to it
demoData = MyDemoData.Instance;
// Use demo config or regular code path?
if (demoData != null)
{
Debug.Log($"Demo configuration found. User Name: {demoData.UserName}");
}
else
{
Debug.Log("No demo configuration found. Using regular app flow.");
}
}
Caveats and Disclaimers
This project was created as one potential way of solving the demo data problem, but developers should always evaluate if an approach meets their needs. For example, though the configuration file gets compiled into the application, tools may exist to reverse engineer assets stored in a Unity project. If the demo is used in a controlled environment where users don’t have access to binaries, this may be a lesser concern. Still, demo or not, ISVs should always have mechanisms in place to disable user accounts or revoke API keys if that information falls into the wrong hands.